Before we explain step by step about php crud operation using mysqli. Lets understand what is CRUD?
In the world of web development, performing CRUD (Create, Read, Update, Delete) operations is a fundamental task. PHP, a server-side scripting language, provides developers with the tools to interact with databases effectively. One of the popular methods for database interaction in PHP is through MySQLi, which stands for MySQL Improved. In this comprehensive guide, we will walk you through the PHP CRUD operations using MySQLi, enabling you to master the art of handling data with grace and efficiency.
Table of Contents
Introduction to PHP CRUD Operations using MySQLi
Before diving into the specifics of PHP and MySQLi, let’s take a moment to understand what CRUD operations are and why they are essential in web development.
What Are CRUD Operations?
CRUD operations are the four basic functions that are essential to any application that involves data manipulation. They are:
- Create: The ‘C’ in CRUD stands for Create, which involves adding new records to your database.
- Read: The ‘R’ stands for Read, which means fetching and displaying data from your database.
- Update: ‘U’ refers to Update, allowing you to modify existing records in your database.
- Delete: Lastly, ‘D’ represents Delete, enabling the removal of records from the database.
These operations are the building blocks of any dynamic web application and we will explore it in more detail in the below article for php crud operation using mysqli.

Connecting to the Database
To work with a MySQL database in PHP, you must establish a connection. Use the following code snippet:
phpCopy code<?php
$host = "your_host";
$username = "your_username";
$password = "your_password";
$database = "your_database";
$mysqli = new mysqli($host, $username, $password, $database);
if ($mysqli->connect_error) {
die("Connection failed: " . $mysqli->connect_error);
}
?>
Ensure you replace placeholders with your actual database credentials.
These operations are the backbone of every dynamic web application, from a simple blog to a complex e-commerce site. Hence when developing php crud operation using mysqli follow the below steps.
Setting up Your Development Environment
Before you can start performing CRUD operations, you need to set up your development environment. Here are the steps to get you started.
Step 1: Install PHP
To begin, you need to have PHP installed on your system. You can download the latest version of PHP from the official website (https://www.php.net/downloads.php) and follow the installation instructions provided for your specific operating system.
Step 2: Install a Web Server
To test your PHP code locally, you’ll also need a web server. Popular options include Apache, Nginx, or XAMPP (which includes Apache, MySQL, PHP, and Perl). Install one of these servers according to your preferences and system requirements.
Step 3: Install MySQL
MySQL is a widely used relational database management system. You can download it from the official website (https://www.mysql.com/downloads/) and follow the installation instructions.
Step 4: Set Up a Database
Create a database that you will use for your CRUD operations. You can use tools like phpMyAdmin or MySQL Workbench to create a new database and tables. An alternative and better way to generate a database is by using the PHP Code Builder where using a simple form you can name the fields and select the type of input easily. Our simple PHP Code builder will generate the SQL file with the variable types along with 2 default fields id & status to every database. It also generates the complete php crud operation using mysqli. So you can download the code files automatically by filling out a simple form.
Connecting to the Database
To work with a MySQL database in PHP, you must establish a connection. Use the following code snippet:
<?php
$host = "your_host";
$username = "your_username";
$password = "your_password";
$database = "your_database";
$mysqli = new mysqli($host, $username, $password, $database);
if ($mysqli->connect_error) {
die("Connection failed: " . $mysqli->connect_error);
}
?>
Ensure you replace placeholders with your actual database credentials.
PHP CRUD Operation Using MySQLi
With your development environment in place, it’s time to start performing CRUD operations with PHP and MySQLi. You can even use PHP Code Builder for crud operation in php.
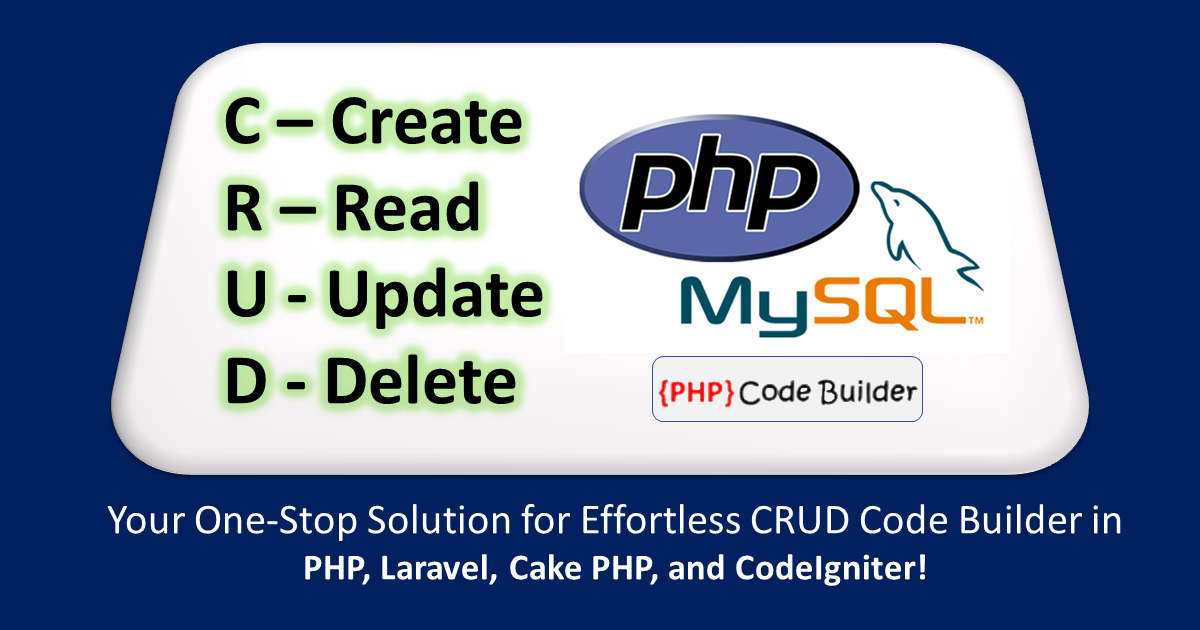
Understanding MySQLi for Database Connectivity
This is a critical section in our comprehensive guide on PHP CRUD operations using MySQLi. In this section, we delve into the fundamentals of MySQLi and its role in establishing a robust connection to a MySQL database. This knowledge is essential for anyone aiming to develop web applications that interact with a database effectively using PHP CRUD Operation Using MySQLi.
- Introduction to MySQLi: We provide an overview of MySQLi, explaining its significance in modern web development. We discuss the differences between MySQLi and the older MySQL extension and why MySQLi is the preferred choice.
- Configuring Database Connection: Here, we walk readers through the process of configuring a connection to a MySQL database using MySQLi. We cover details such as host, username, password, and database selection.
- Error Handling: Understanding how to handle errors when establishing a database connection is crucial. We discuss error reporting and handling techniques to ensure smooth connectivity.
- Performing a Test Connection: We guide users on how to test their database connection to verify that it’s set up correctly before proceeding with CRUD operations.
- Best Practices: We provide tips and best practices for securing and optimizing the database connection for better performance and security.
By the end of this section, readers will have a solid grasp of PHP CRUD Operation Using MySQLi and be well-prepared to start working with databases in PHP, a fundamental skill in web development.
Creating the ‘C’ in CRUD: Adding Data
While working on php crud operation using mysqli. The create operation allows you to insert new records into the database. Here’s a simple example of how to add a new record using PHP and MySQLi:
<?php
// Establish a database connection
$connection = new mysqli("localhost", "username", "password", "database_name");
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
// Data to be inserted
$name = "John Doe";
$email = "john@example.com";
// SQL query to insert data
$sql = "INSERT INTO users (name, email) VALUES ('$name', '$email')";
if ($connection->query($sql) === TRUE) {
echo "New record created successfully";
} else {
echo "Error: " . $sql . "<br>" . $connection->error;
}
$connection->close();
?>
Reading Data: The ‘R’ in CRUD
The read operation retrieves data from the database when you work on php crud operation using mysqli. You can use SQL SELECT statements to retrieve data based on your criteria. Here’s an example:
<?php
$connection = new mysqli("localhost", "username", "password", "database_name");
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
// SQL query to retrieve data
$sql = "SELECT * FROM users";
$result = $connection->query($sql);
if ($result->num_rows > 0) {
while ($row = $result->fetch_assoc()) {
echo "ID: " . $row["id"] . " - Name: " . $row["name"] . " - Email: " . $row["email"] . "<br>";
}
} else {
echo "0 results";
}
$connection->close();
?>

Updating Records: Mastering the ‘U’ in CRUD
The update operation allows you to modify existing records in the database while writing code for php crud operation using mysqli. Here’s a simple example:
<?php
$connection = new mysqli("localhost", "username", "password", "database_name");
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
// Data to be updated
$id = 1;
$newName = "Jane Doe";
// SQL query to update data
$sql = "UPDATE users SET name='$newName' WHERE id=$id";
if ($connection->query($sql) === TRUE) {
echo "Record updated successfully";
} else {
echo "Error updating record: " . $connection->error;
}
$connection->close();
?>
Deleting Data: The ‘D’ in CRUD Operations
The delete operation removes records from the database when you code php crud operation using mysqli. Here’s an example of how to delete a record using PHP and MySQLi:
<?php
$connection = new mysqli("localhost", "username", "password", "database_name");
if ($connection->connect_error) {
die("Connection failed: " . $connection->connect_error);
}
// Data to be deleted
$id = 1;
// SQL query to delete data
$sql = "DELETE FROM users WHERE id=$id";
if ($connection->query($sql) === TRUE) {
echo "Record deleted successfully";
} else {
echo "Error deleting record: " . $connection->error;
}
$connection->close();
?>
PHP Code Builder is a user-friendly no code builder online platform designed to make CRUD (Create, Read, Update, Delete) operations a breeze for developers working with PHP, Laravel, Cake PHP, and Codeigniter. Our mission is to streamline and simplify the often repetitive and time-consuming task of building database-driven applications. See it in action in the below video for php crud operation using mysqli :-
The same tool can be used for laravel crud code generation or crud operation in laravel. CRUD operation in php, crud in php with mysql.
Security Measures for PHP CRUD Development
Security is paramount in PHP CRUD (Create, Read, Update, Delete) development to protect your application and its data. Here are 5 crucial security measures to implement in php crud operation using mysqli:
- Input Validation and Sanitization:
- Ensure that all user inputs, whether from forms or external sources, are thoroughly validated and sanitized. Use functions like
filter_input()
and input validation libraries to prevent SQL injection and cross-site scripting (XSS) attacks.
- Ensure that all user inputs, whether from forms or external sources, are thoroughly validated and sanitized. Use functions like
- Prepared Statements:
- When interacting with your database, use prepared statements and parameterized queries to avoid SQL injection. This practice ensures that user-supplied data is treated as data and not executable SQL code.
- Authentication and Authorization:
- Implement robust user authentication to control access to CRUD operations. Users should only be able to perform actions for which they have proper authorization. Use role-based access control (RBAC) to manage user permissions effectively.
- Data Encryption:
- Encrypt sensitive data, such as passwords and personal information, both in transit and at rest. Employ secure encryption protocols like HTTPS for data transmission and utilize bcrypt or other strong encryption methods for stored data.
- Session Management and Cross-Site Request Forgery (CSRF) Protection:
- Secure your application against session hijacking and CSRF attacks. Use secure session management techniques, regenerate session IDs after successful login, and implement anti-CSRF tokens to verify the authenticity of requests.
By incorporating these security measures into your PHP CRUD development, you can significantly reduce the risk of common web application vulnerabilities and protect your application and its data from malicious threats. Thus, while developing a PHP CRUD operation using mysqli you can implement the above suggestions.
Optimizing Performance for CRUD Applications
Optimizing the performance of CRUD (Create, Read, Update, Delete) applications is crucial for providing a seamless user experience. Here are some tips to enhance the performance of your php crud operation using mysqli :
- Database Indexing:
- Properly index your database tables to speed up data retrieval. Identify the columns frequently used in WHERE clauses and apply appropriate indexes. However, avoid over-indexing, as it can lead to performance issues.
- Caching:
- Implement caching mechanisms to reduce the load on your database. Consider using in-memory caches like Redis or Memcached to store frequently accessed data. Caching can significantly boost read-heavy CRUD operations.
- Query Optimization:
- Optimize your SQL queries to reduce execution time. Use efficient query design, avoid SELECT * statements, and retrieve only the necessary data. Profile and analyze queries to identify and eliminate bottlenecks.
- Pagination:
- Implement pagination for large result sets to limit the number of records retrieved in a single query. This reduces the strain on your database and improves the response time.
- Asynchronous Processing:
- Offload resource-intensive tasks, such as sending emails or generating reports, to asynchronous processes. This keeps your php crud operation using mysqli responsive and scalable.

Advanced Techniques and Best Practices for php crud operation using mysqli
Certainly! Here is a list of advanced techniques and best practices for PHP CRUD operations using MySQLi:
- Prepared Statements: Utilize prepared statements to protect against SQL injection attacks and enhance query performance. This technique involves binding parameters to your queries, making them more secure and efficient. Hence when developing php crud operation using mysqli its advised to use prepared statements.
- Transaction Management: Implement database transactions to ensure data integrity. Transactions help maintain consistency in the database by allowing a group of queries to be executed as a single unit, either entirely or not at all.
- Database Indexing: Understand the importance of database indexing to optimize CRUD operations. Proper indexing can significantly speed up data retrieval and modification.
- Data Validation: Apply robust data validation techniques to ensure that only valid and expected data is entered into the database. This reduces the likelihood of errors and security vulnerabilities.
- Error Handling and Logging: Set up comprehensive error handling and logging mechanisms to detect issues promptly and troubleshoot problems effectively. Detailed error messages should not be exposed to users but should be logged for administrators.
- User Authentication and Authorization: Integrate user authentication and authorization to control who can perform CRUD operations on your application. Implement role-based access control to limit access to specific functionalities.
- Caching Strategies: Implement caching mechanisms to reduce the load on the database server, especially for read-heavy CRUD operations. Techniques like Memcached or Redis can be valuable.
- Normalization and Denormalization: Understand when to normalize and when to denormalize your database schema. This decision can impact performance and storage efficiency.
- API Development: Consider building a well-defined API for your CRUD operations, allowing for easy integration with other systems or platforms. RESTful APIs are a common choice for web applications.
- Scalability Planning: Plan for scalability from the outset. Design your database and codebase in a way that allows for horizontal or vertical scaling as your application grows.
- Testing and Test Automation: Create a comprehensive testing strategy that covers unit testing, integration testing, and end-to-end testing for your CRUD operations. Implement test automation to catch regressions.
- Documentation: Maintain clear and up-to-date documentation for your CRUD operations, APIs, and database schema. This helps both developers and users understand how to interact with your application.
- Regular Backups: Implement automated and regular backup procedures to protect your data against loss or corruption.
- Monitoring and Performance Tuning: Use monitoring tools to keep an eye on the performance of your CRUD operations. Continuously optimize queries and database configurations for better speed and efficiency.
- Compliance and Security: Stay up-to-date with data protection laws and security best practices. Implement encryption and other security measures to safeguard sensitive data.
By following these advanced techniques and best practices, you can create robust and high-performing PHP CRUD operations using MySQLi while ensuring data integrity and security.

Conclusion for developing php crud operation using mysqli
In this guide, we’ve covered the basics of PHP CRUD operations using MySQLi. You’ve learned how to set up your development environment, perform create, read, update, and delete operations, and work with databases effectively. Armed with this knowledge, you’re well on your way to building dynamic and data-driven web applications with PHP and MySQLi.
Remember that practice makes perfect, and the more you work with CRUD operations, the more proficient you’ll become. So, roll up your sleeves, dive into the code, and start creating amazing web applications with PHP and MySQLi to develop php crud operation using mysqli.
FAQ for php crud operation using mysqli
What is MySQLi?
MySQLi stands for MySQL Improved. It’s a database driver for PHP that provides enhanced features and security when interacting with MySQL databases. MySQLi is an improved version of the original MySQL extension for PHP, offering better performance and support for modern MySQL features.
Can I use MySQL instead of MySQLi?
You can, but MySQLi is recommended due to its improved features, better security, and support for prepared statements. While you can use the older MySQL extension, it’s advisable to use MySQLi for modern web development.
What are prepared statements?
Prepared statements are a way to execute SQL queries securely by separating SQL code from user input, preventing SQL injection. Prepared statements help protect your database from malicious attacks, making them a best practice in web development.
Is it necessary to sanitize user input in CRUD operations?
Yes, it’s essential to validate and sanitize user input to prevent security vulnerabilities and ensure data integrity in your database. Proper input validation is a critical security measure to safeguard your application against potential threats.